Makefile
The preferred way (compulsory if you are contributing to the core of the library) to build MARTe2 based projects is to follow the MARTe2 Makefile structure.
All the Makefiles share a common Makefile.inc which in turn imports the main definitions from the operating system and architecture MakeDefaults.
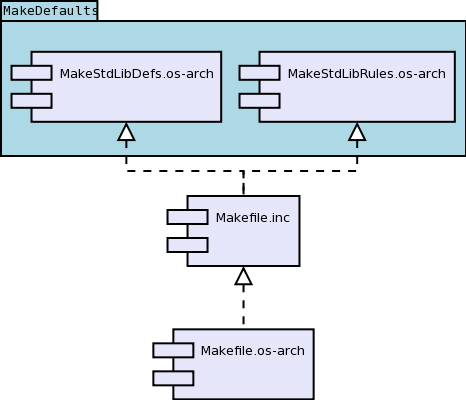
The definitions and the Makefile rules for the selected operating system and architecture will have to exist in the MakeDefaults folder.
The Makefile.inc defines all the rules that are common to all the operating systems and architecture. The Makefile.os-arch (where os.arch is the selected operating systems/architecture) shall include, as a minimum, the Makefile.inc and shall define the TARGET
operating system/architecture (if not already defined as a system property).
TARGET=os-arch
include Makefile.inc
Where os-arch is the selected operating systems/architecture (i.e. the value of the TARGET
environment variable).
As an example, a Makefile to compile for linux on an armv8 (i.e. Makefile.armv8-linux
) could look like:
TARGET=armv8-linux
include Makefile.inc
#Example of an operating system/architecture specific flag
LIBRARIES += -lm
Note
The Makefile.gcc
allows to recycle the same Makefile for several gcc
based projects. The Makefile.linux
in the root folder of MARTe2 assumes TARGET=x86-linux
. For gcc projects in other operating systems/architectures, make sure to export the appropriate architecture before executing make -f Makefile.gcc
.
Definitions
The following are definitions from the MakeDefaults/MakeStdLibDefs.os-arch file and may be either complemented or overridden by a project specific Makefile.inc or by a project specific Makefile.os-arch.
Acronym |
Definition |
---|---|
ARCHITECTURE |
The architecture being compiled (e.g. x86_gcc). |
BUILD_DIR |
Default location where the compiled output files are written into. The default rule is: |
CFLAGS |
Compiler flags specific to C unit files (e.g. -fPIC). |
CPPFLAGS |
Compiler flags specific to C++ unit files (e.g. -frtti). |
DEBUG |
Debug related flags (e.g. -g). |
ENVIRONMENT |
The operating system environment being compiled (e.g. Linux). |
INCLUDES |
Folders to be included during the compilation process. |
LIBRARIES |
Shared libraries to be used during the linking process (e.g. -lm). |
LIBRARIES_STATIC |
Static libraries to be used during the linking process. |
MAKEDEFAULTDIR |
The location of the MakeDefault directory. |
PACKAGE |
Name of the package to which the output belongs to (can be overriden by BUILD_DIR). |
OBJSX |
List of unit files to be compiled. The format is NAME_OF_FILE.x |
OPTIM |
Optimisation related flags (e.g. -O2) |
ROOT_DIR |
Location of the Build directory (can be overriden by BUILD_DIR). |
SPB |
List of other subfolders to be compiled. The format is NAME_OF_FOLDER.x |
Supported outputs
The Makefile.inc also defines the type of outputs that are to be produced. The following outputs are supported.
Acronym |
Definition |
---|---|
ASMEXT |
Assembly file. |
DLLEXT |
Shared library. |
DRVEXT |
Driver file. |
EXEEXT |
Executable. |
GAMEXT |
GAM file. |
LIBEXT |
Static library. |
OBJEXT |
Object file. |
An example of Makefile.inc file is:
#List of objects to be compiled
OBJSX=File1.x \
File2.x \
File3.x
#Name of the package (see the Definitions above)
PACKAGE=Components/Examples
#Where is the Build directory
ROOT_DIR=../../../../
#Compulsory. Defines the location of the MakeDefaults directory. MARTe2_DIR is the location where MARTe2 is installed.
MAKEDEFAULTDIR=$(MARTe2_DIR)/MakeDefaults
include $(MAKEDEFAULTDIR)/MakeStdLibDefs.$(TARGET)
#List of folders to be included
INCLUDES += -I.
INCLUDES += -I$(MARTe2_DIR)/Source/Core/BareMetal/L0Types
INCLUDES += -I$(MARTe2_DIR)/Source/Core/BareMetal/L1Portability
INCLUDES += -I$(MARTe2_DIR)/Source/Core/BareMetal/L2Objects
INCLUDES += -I$(MARTe2_DIR)/Source/Core/BareMetal/L3Streams
INCLUDES += -I$(MARTe2_DIR)/Source/Core/BareMetal/L4Messages
INCLUDES += -I$(MARTe2_DIR)/Source/Core/BareMetal/L4Configuration
INCLUDES += -I$(MARTe2_DIR)/Source/Core/BareMetal/L5GAMs
INCLUDES += -I$(MARTe2_DIR)/Source/Core/Scheduler/L1Portability
INCLUDES += -I$(MARTe2_DIR)/Source/Core/Scheduler/L3Services
INCLUDES += -I$(MARTe2_DIR)/Source/Core/Scheduler/L4Messages
#Outputs to be generated: a static library and a shared library in this example
all: $(OBJS) $(SUBPROJ) \
$(BUILD_DIR)/MYLIB1$(LIBEXT) \
$(BUILD_DIR)/MYLIB1$(DLLEXT)
echo $(OBJS)
include $(MAKEDEFAULTDIR)/MakeStdLibRules.$(TARGET)
Note that if the output file is also a unit file (i.e. if a .c or .cpp also exist) it shall not be declared in the OBJSX section (see example below).
In the case of executables and shared libraries, a unit file with the name of the output file shall exist (i.e. if an executable named Main.ex is to be created, a file named Main.cpp shall exist).
Nested compilation
Using the SPB
definition the Makefile will compile several folders in the provided sequence.
For example, the instruction SPB=FOLDER1.x FOLDER2/FOLDER3.x
would trigger the compilation in FOLDER1 and in FOLDER2/FOLDER3.
Note that inside each of these folders a Makefile.inc and one, or more, Makefile.os-arch files will have to exist.
Example
The following example builds a library using the files File1.cpp and File2.cpp. Note that the File1.cpp is not included in the OBJSX since it is also the name of the executable to be generated.
1#############################################################
2#
3# Copyright 2015 F4E | European Joint Undertaking for ITER
4# and the Development of Fusion Energy ('Fusion for Energy')
5#
6# Licensed under the EUPL, Version 1.1 or - as soon they
7# will be approved by the European Commission - subsequent
8# versions of the EUPL (the "Licence");
9# You may not use this work except in compliance with the
10# Licence.
11# You may obtain a copy of the Licence at:
12#
13# http://ec.europa.eu/idabc/eupl
14#
15# Unless required by applicable law or agreed to in
16# writing, software distributed under the Licence is
17# distributed on an "AS IS" basis,
18# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
19# express or implied.
20# See the Licence for the specific language governing
21# permissions and limitations under the Licence.
22#
23#############################################################
24
25#Named of the unit files to be compiled
26OBJSX=ExFile2.x
27
28#Create a folder named Examples in the Build directory
29PACKAGE=Examples
30
31#Location of the Build directory
32ROOT_DIR=../../../../../..
33
34#Location of the MakeDefaults directory.
35#Note that the ROOT_DIR environment variable
36#must have been exported before
37MARTe2_MAKEDEFAULT_DIR?=$(ROOT_DIR)/MakeDefaults
38
39include $(MARTe2_MAKEDEFAULT_DIR)/MakeStdLibDefs.$(TARGET)
40
41INCLUDES += -I$(ROOT_DIR)/Source/Core/BareMetal/L0Types
42INCLUDES += -I$(ROOT_DIR)/Source/Core/BareMetal/L1Portability
43INCLUDES += -I$(ROOT_DIR)/Source/Core/BareMetal/L2Objects
44INCLUDES += -I$(ROOT_DIR)/Source/Core/BareMetal/L3Streams
45INCLUDES += -I$(ROOT_DIR)/Source/Core/BareMetal/L4Configuration
46INCLUDES += -I$(ROOT_DIR)/Source/Core/BareMetal/L4Messages
47INCLUDES += -I$(ROOT_DIR)/Source/Core/BareMetal/L5GAMs
48INCLUDES += -I$(ROOT_DIR)/Source/Core/FileSystem/L1Portability
49INCLUDES += -I$(ROOT_DIR)/Source/Core/FileSystem/L3Streams
50INCLUDES += -I$(ROOT_DIR)/Source/Core/Scheduler/L1Portability
51INCLUDES += -I$(ROOT_DIR)/Source/Core/Scheduler/L3Services
52INCLUDES += -I$(ROOT_DIR)/Source/Core/Scheduler/L4Messages
53INCLUDES += -I$(ROOT_DIR)/Source/Core/Scheduler/L4StateMachine
54
55#Generate an executable named ExFile1$(EXEEXT).
56#Note that the file ExFile1.cpp has to exist and must
57#not be declared in the OBJSX list.
58all: $(OBJS) $(SUBPROJ) \
59 $(BUILD_DIR)/ExFile1$(EXEEXT)
60 echo $(OBJS)
61
62include depends.$(TARGET)
63
64include $(MARTe2_MAKEDEFAULT_DIR)/MakeStdLibRules.$(TARGET)
1#############################################################
2#
3# Copyright 2015 EFDA | European Joint Undertaking for ITER
4# and the Development of Fusion Energy ("Fusion for Energy")
5#
6# Licensed under the EUPL, Version 1.1 or - as soon they
7# will be approved by the European Commission - subsequent
8# versions of the EUPL (the "Licence");
9# You may not use this work except in compliance with the
10# Licence.
11# You may obtain a copy of the Licence at:
12#
13# http://ec.europa.eu/idabc/eupl
14#
15# Unless required by applicable law or agreed to in
16# writing, software distributed under the Licence is
17# distributed on an "AS IS" basis,
18# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
19# express or implied.
20# See the Licence for the specific language governing
21# permissions and limitations under the Licence.
22#
23# $Id: Makefile.gcc 3 2015-01-15 16:26:07Z aneto $
24#
25#############################################################
26
27#Defines the target architecture
28export TARGET=x86-linux
29
30include Makefile.inc
31
32#Must link with the MARTe2 library
33LIBRARIES += -L$(ROOT_DIR)/Build/$(TARGET)/Core -lMARTe2
To try the example execute:
export MARTe2_DIR=FOLDER_WHERE_MARTe2_IS_INSTALLED
cd $MARTe2_DIR/Docs/User/source/_static/examples/Makefiles
make -f Makefile.x86-linux
export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_DIR/Build/x86-linux/Core/
../../../../../../Build/x86-linux/Examples/Makefiles/ExFile1.ex