Objects¶
The MARTe2 Object is the root class of the framework and offers the following features:
Automatic life cycle management using a smart pointer mechanism (see References);
Data driven construction (in runtime) using the class name;
Standard initialisation and configuration interface (more information here);
Standard messaging interface (more information here);
Allocation on a user selectable heap.
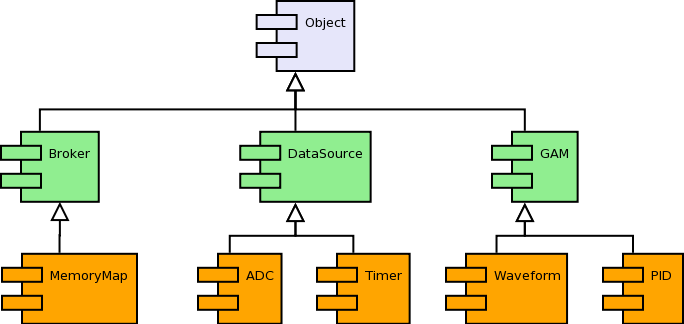
ClassRegistryDatabase¶
In order to automatically register a MARTe Object into the ClassRegistryDatabase, the following macros must be used:
CLASS_REGISTER_DECLARATION()
in the class declaration and;CLASS_REGISTER(NAME_OF_THE_CLASS)
in the class definition, where NAME_OF_THE_CLASS is the name of the class where theCLASS_REGISTER_DECLARATION()
has been declared;
class ControllerEx1: public MARTe::Object {
public:
/**
* Compulsory for the class to be automatically registered.
*/
CLASS_REGISTER_DECLARATION()
ControllerEx1() {
}
//Any other class methods
...
};
//Usually declared in the .cpp unit file.
CLASS_REGISTER(ControllerEx1, "")
When a MARTe application starts (or when one of the shared libraries is explicitly opened, see the configuration Class= parameter here ), these macros will force the relevant class to register in the ClassRegistryDatabase.
Note
The underlying mechanism is based on the fact that these macros create a ClassRegistryItem global static variable (per class type) which will then automatically add (i.e. register) itself to the class database (see Add in ClassRegistryDatabase).
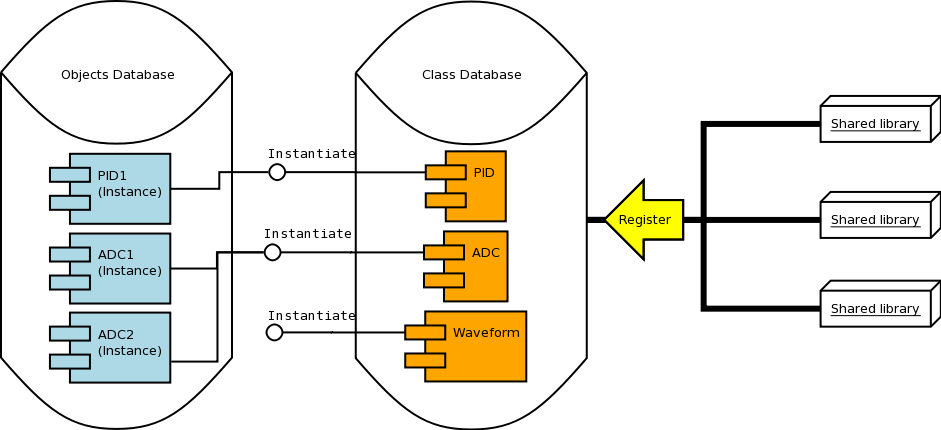
Once the class is registered the database can be queried and new object instances created.
Examples¶
The following examples show how to query the contents of a ClassRegistryDatabase…
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 | /**
* @file ObjectsExample1.cpp
* @brief Source file for class ObjectsExample1
* @date 14/03/2018
* @author Andre' Neto
*
* @copyright Copyright 2015 F4E | European Joint Undertaking for ITER and
* the Development of Fusion Energy ('Fusion for Energy').
* Licensed under the EUPL, Version 1.1 or - as soon they will be approved
* by the European Commission - subsequent versions of the EUPL (the "Licence")
* You may not use this work except in compliance with the Licence.
* You may obtain a copy of the Licence at: http://ec.europa.eu/idabc/eupl
*
* @warning Unless required by applicable law or agreed to in writing,
* software distributed under the Licence is distributed on an "AS IS"
* basis, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the Licence permissions and limitations under the Licence.
* @details This source file contains the definition of all the methods for
* the class ObjectsExample1 (public, protected, and private). Be aware that some
* methods, such as those inline could be defined on the header file, instead.
*/
#define DLL_API
/*---------------------------------------------------------------------------*/
/* Standard header includes */
/*---------------------------------------------------------------------------*/
/*---------------------------------------------------------------------------*/
/* Project header includes */
/*---------------------------------------------------------------------------*/
#include "AdvancedErrorManagement.h"
#include "ClassRegistryDatabase.h"
#include "ErrorLoggerExample.h"
#include "Object.h"
#include "StreamString.h"
/*---------------------------------------------------------------------------*/
/* Static definitions */
/*---------------------------------------------------------------------------*/
/*---------------------------------------------------------------------------*/
/* Method definitions */
/*---------------------------------------------------------------------------*/
namespace MARTe2Tutorial {
/**
* @brief A MARTe::Object class will be automatically registered
* into the ClassRegistryDatabase.
*/
class ControllerEx1: public MARTe::Object {
public:
CLASS_REGISTER_DECLARATION()
/**
* @brief NOOP.
*/
ControllerEx1 () {
gain = 0u;
}
/**
* A property.
*/
MARTe::uint32 gain;
};
CLASS_REGISTER(ControllerEx1, "")
}
int main(int argc, char **argv) {
using namespace MARTe;
SetErrorProcessFunction(&ErrorProcessExampleFunction);
//List all the classes that have been registered.
uint32 i;
ClassRegistryDatabase *crdSingleton = ClassRegistryDatabase::Instance();
uint32 numberOfClasses = crdSingleton->GetSize();
for (i = 0u; i < numberOfClasses; i++) {
const ClassRegistryItem *classRegistryItem = crdSingleton->Peek(i);
const ClassProperties *classProperties = classRegistryItem->GetClassProperties();
CCString className = classProperties->GetName();
uint14 classUID = classProperties->GetUniqueIdentifier();
REPORT_ERROR_STATIC(ErrorManagement::Information, "Counter: %d | "
"Class: %s | UID : %d", i, className.GetList(), classUID);
}
//Find the ControllerEx1 class
CCString classNameToSearch = "ControllerEx1";
const ClassRegistryItem *classRegistryItem = crdSingleton->Find(classNameToSearch);
if (classRegistryItem != NULL) {
const ClassProperties *classProperties = classRegistryItem->GetClassProperties();
CCString className = classProperties->GetName();
uint14 classUID = classProperties->GetUniqueIdentifier();
REPORT_ERROR_STATIC(ErrorManagement::Information, "Found class: %s |"
" UID : %d", className.GetList(), classUID);
}
else {
REPORT_ERROR_STATIC(ErrorManagement::FatalError, "Could not find class "
"with name: %s", classNameToSearch.GetList());
}
return 0;
}
|
… and how to instantiate a new object. Note that the Build method requires as an input an HeapI implementation (this is particularly useful for embedded systems).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 | /**
* @file ObjectsExample2.cpp
* @brief Source file for class ObjectsExample2
* @date 14/03/2018
* @author Andre' Neto
*
* @copyright Copyright 2015 F4E | European Joint Undertaking for ITER and
* the Development of Fusion Energy ('Fusion for Energy').
* Licensed under the EUPL, Version 1.1 or - as soon they will be approved
* by the European Commission - subsequent versions of the EUPL (the "Licence")
* You may not use this work except in compliance with the Licence.
* You may obtain a copy of the Licence at: http://ec.europa.eu/idabc/eupl
*
* @warning Unless required by applicable law or agreed to in writing,
* software distributed under the Licence is distributed on an "AS IS"
* basis, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the Licence permissions and limitations under the Licence.
* @details This source file contains the definition of all the methods for
* the class ObjectsExample2 (public, protected, and private). Be aware that some
* methods, such as those inline could be defined on the header file, instead.
*/
#define DLL_API
/*---------------------------------------------------------------------------*/
/* Standard header includes */
/*---------------------------------------------------------------------------*/
/*---------------------------------------------------------------------------*/
/* Project header includes */
/*---------------------------------------------------------------------------*/
#include "AdvancedErrorManagement.h"
#include "ClassRegistryDatabase.h"
#include "ErrorLoggerExample.h"
#include "Object.h"
#include "StreamString.h"
/*---------------------------------------------------------------------------*/
/* Static definitions */
/*---------------------------------------------------------------------------*/
/*---------------------------------------------------------------------------*/
/* Method definitions */
/*---------------------------------------------------------------------------*/
namespace MARTe2Tutorial {
/**
* @brief A MARTe::Object class will be automatically registered
* into the ClassRegistryDatabase.
*/
class ControllerEx1: public MARTe::Object {
public:
CLASS_REGISTER_DECLARATION()
/**
* @brief NOOP.
*/
ControllerEx1() {
gain = 0xffu;
}
/**
* A property.
*/
MARTe::uint32 gain;
};
CLASS_REGISTER(ControllerEx1, "")
}
int main(int argc, char **argv) {
using namespace MARTe;
using namespace MARTe2Tutorial;
SetErrorProcessFunction(&ErrorProcessExampleFunction);
//Find the ControllerEx1 class
ClassRegistryDatabase *crdSingleton = ClassRegistryDatabase::Instance();
CCString classNameToSearch = "ControllerEx1";
const ClassRegistryItem *classRegistryItem = crdSingleton->Find(classNameToSearch);
if (classRegistryItem != NULL) {
//Get the object builder (which knows how to build classes of this type).
const ObjectBuilder *builder = classRegistryItem->GetObjectBuilder();
if (builder != NULL) {
//Build the class using the specified memory heap
Object *obj = builder->Build(GlobalObjectsDatabase::Instance()->GetStandardHeap());
if (obj != NULL) {
ControllerEx1 *obj2 = dynamic_cast<ControllerEx1 *>(obj);
if (obj2 != NULL) {
obj2->SetName("ControllerInstance1");
REPORT_ERROR_STATIC(ErrorManagement::Information, "Successfully managed "
"to create an instance of: %s and to set "
"its name to %s", classNameToSearch.GetList(), obj2->GetName());
}
}
}
else {
REPORT_ERROR_STATIC(ErrorManagement::FatalError, "Could not find the ObjectBuilder "
"for the class with name: %s", classNameToSearch.GetList());
}
}
else {
REPORT_ERROR_STATIC(ErrorManagement::FatalError, "Could not find class "
"with name: %s", classNameToSearch.GetList());
}
return 0;
}
|
Instructions on how to compile and execute the examples can be found here.